Deze tutorial is overbodig geworden door de mogelijkheden die aan de loc zijn toegevoegd door het DCR&MarkA Repaint Pack | This tutorial has become obsolete due to the added possibilities in the loco by the DCR&MarkA Repaint Pack |
![]() |
![]() |
Wat is er realistischer dan een IC Berlijn met 17xx ervoor die het station binnen komt en na stilstand de tweede stroomafnemer tegen de draad zet? Of bij een DB 16xx met staaltrein of RRF 44xx met keteltrein wanneer ze onderweg een tussenstop maken. LUA scripting maakt dit allemaal mogelijk! Zowel bij de spelerstrein als ook AI treinen! |
What would be more realistic than an IC Berlin with 17xx loco raising it's second pantograph after having stopped at a station? Or a DB 16xx with a steel train or RRF 44xx with a tanker train doing that when having to stopped along the way. LUA scripting makes all that possible! As well with the players train as with AI trains too! |
Voor meer informatie over hoe en wat met LUA scripting verwijs ik je graag naar elders. Matt Paddlesden heeft een uitstekend filmpje op YouTube en daaruit gemaakte blogs voor degene die zich willen verdiepen in de beginselen van LUA scripting in scenario's. |
For more information about the basics of LUA scripting I'd like to point you to somewhere else. Matt Paddlesden has an excelent video on YouTube and created blogs from that for everyone who want to learn the basics of LUA scripting in scenarios. |
Ik beperkt me hier alleen specifiek op de LUA scripting voor de stroomafnemers. | Here I'll confine myself only to LUA scripting for the pantographs. |
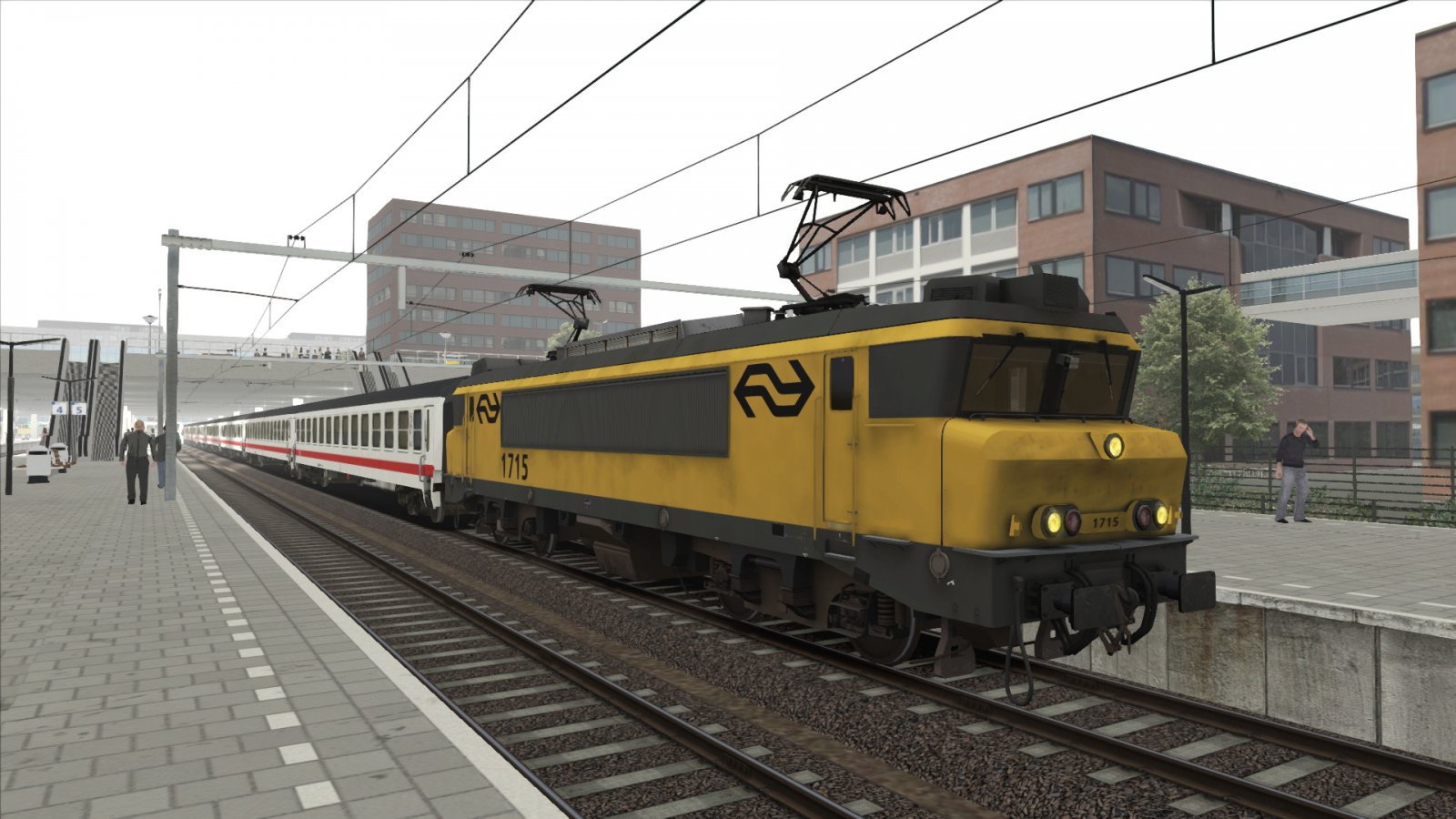
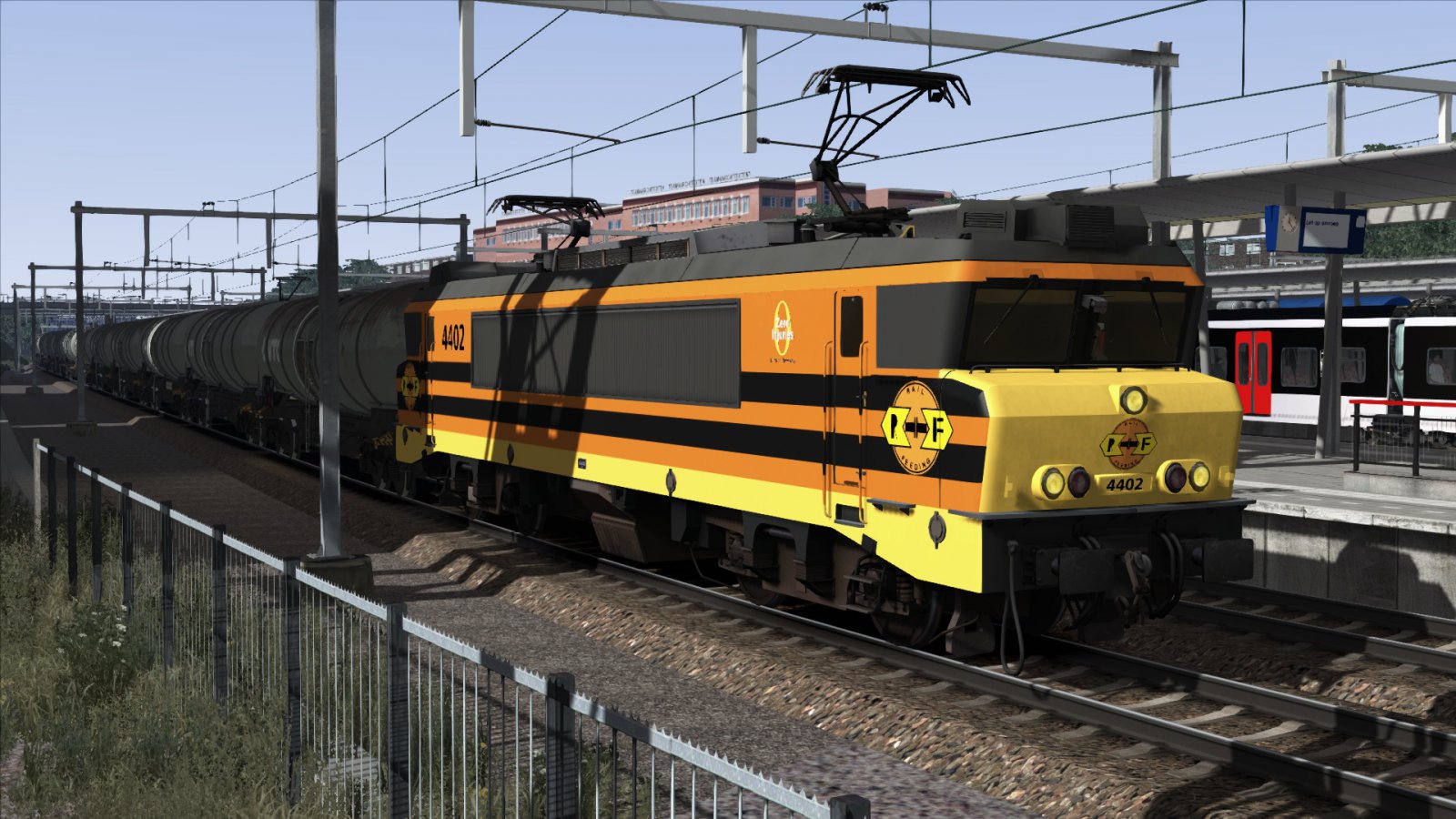
Begin het LUA script met het declareren van enkele variabelen: | Start the LUA script with the declaration of some variables: |
Code: Selecteer alles
-- true/false defn
FALSE = 0
TRUE = 1
-- condition return values
CONDITION_NOT_YET_MET = 0
CONDITION_SUCCEEDED = 1
CONDITION_FAILED = 2
-- Speed
MPH = 2.23693629
KMH = 3.6
-- Set SpeedUnits
gSpeedUnits = KMH
Plaats in ieder LUA script voor het eerste event dit: | Place this in front of your first event in every LUA script: |
Code: Selecteer alles
function OnEvent(event)
_G["OnEvent" .. event]();
end
Maak vervolgens een event aan: | Then create an event: |
Code: Selecteer alles
function OnEventEventName()
SysCall ( "PlayerEngine:SetControlValue", "Frontpantoswitch", 0, 0 );
SysCall ( "PlayerEngine:SetControlValue", "Backpantoswitch", 0, 1 );
SysCall ( "ScenarioManager:BeginConditionCheck", "ConditionName" );
end
Dit event stelt de basis settings van de stroomafnemers in. Vervolgens wordt er een statuscontrole gestart. |
This event sets the basic settings of the pantographs. Next it starts a condition check. |
Plaats in ieder LUA script voor de eerste condition dit: | Place this in front of your first condition in every LUA script: |
Code: Selecteer alles
function TestCondition(condition)
_G["TestCondition" .. condition]();
end
Maak vervolgens een condition aan: | Then create a condition: |
Code: Selecteer alles
function TestConditionConditionName()
speed = math.abs ( ( SysCall ( "PlayerEngine:GetSpeed" ) ) * gSpeedUnits );
if ( speed < 0.1 ) then
SysCall ( "ScenarioManager:TriggerDeferredEvent", "EventName2", 3 );
return CONDITION_SUCCEEDED;
end
return CONDITION_NOT_YET_MET;
end
De conditie controleert de snelheid waarmee de trein rijdt. Wanneer de gewenste conditie is behaald (<0.1 km/u) wordt er na 3 seconden een nieuw event aangeroepen. |
The condition checks the train's speed. When the desired speed (<0.1 km/h) is achieved another event is called after 3 seconds. |
Maak vervolgens een tweede event aan: | Then create a second event: |
Code: Selecteer alles
function OnEventEventName2()
SysCall ( "PlayerEngine:SetControlValue", "Frontpantoswitch", 0, 1 );
SysCall ( "ScenarioManager:BeginConditionCheck", "ConditionName2" );
end
Dit event zet de voorste stroomafnemers tegen de draad. Daarna wordt er een nieuwe statuscontrole gestart. |
This event raises the front pantographs. After that it starts a new condition check. |
Code: Selecteer alles
function TestConditionConditionName2()
speed = math.abs ( ( SysCall ( "PlayerEngine:GetSpeed" ) ) * gSpeedUnits );
if ( speed > 30 ) then
SysCall ( "ScenarioManager:TriggerDeferredEvent", "EventName", 1 );
return CONDITION_SUCCEEDED;
end
return CONDITION_NOT_YET_MET;
end
De conditie controleert of de trein harder rijdt dan 30 km/u. Wanneer dat het geval is wordt na 1 seconden het eerste event weer aangroepen. Daarmee wordt de voorste stroomafnemer gestreken en de eerste conditie gestart voor de volgende stop. |
The condition checks if the train goes faster then 30 km/h. When that is the case the first event is called after 1 second. With that the front pantograph is lowered and the first condition is started again for the next stop. |
Voorbeeldscript: | Examplescript: |
Code: Selecteer alles
-- true/false defn
FALSE = 0
TRUE = 1
-- condition return values
CONDITION_NOT_YET_MET = 0
CONDITION_SUCCEEDED = 1
CONDITION_FAILED = 2
-- Speed
MPH = 2.23693629
KMH = 3.6
-- Set SpeedUnits
gSpeedUnits = KMH
function OnEvent(event)
_G["OnEvent" .. event]();
end
function OnEventPantoDown()
SysCall ( "PlayerEngine:SetControlValue", "Frontpantoswitch", 0, 0 );
SysCall ( "PlayerEngine:SetControlValue", "Backpantoswitch", 0, 1 );
SysCall ( "ScenarioManager:BeginConditionCheck", "PantoUp" );
end
function OnEventPantoUp()
SysCall ( "PlayerEngine:SetControlValue", "Frontpantoswitch", 0, 1 );
SysCall ( "ScenarioManager:BeginConditionCheck", "PantoDown" );
end
function TestCondition(condition)
_G["TestCondition" .. condition]();
end
function TestConditionConditionPantoUp()
speed = math.abs ( ( SysCall ( "PlayerEngine:GetSpeed" ) ) * gSpeedUnits );
if ( speed < 0.1 ) then
SysCall ( "ScenarioManager:TriggerDeferredEvent", "PantoUp", 3 );
return CONDITION_SUCCEEDED;
end
return CONDITION_NOT_YET_MET;
end
function TestConditionConditionPantoDown()
speed = math.abs ( ( SysCall ( "PlayerEngine:GetSpeed" ) ) * gSpeedUnits );
if ( speed > 30 ) then
SysCall ( "ScenarioManager:TriggerDeferredEvent", "PantoDown", 1 );
return CONDITION_SUCCEEDED;
end
return CONDITION_NOT_YET_MET;
end
Roep in de editor event "PantoDown" op om de stroomafnemers in te stellen, de achterste op en de voorste neer. Minimaal één seconde na het starten van de trein! Vervolgens wordt conditie check "PantoUp" gestart. Wanneer een snelheid van 0,1 km/u is bereikt wordt 3 seconden later met event "PantoUp" de voorste stroomafnemer tegen de draad gezet en wordt conditie check "PantoDown" gestart. Is de trein weer onderweg? Dan zal bij het behalen van 30 km/u event "PantoDown" wederom gestart, welke de voorste stroomafnemer strijken en wederom conditie check "PantoUp" starten. |
In the editor call event "PantoDown" to set the pantographs, back one up and front down. One second after the train is started minimal! Then condition check "PantoUp" is started. When the train reaches a speed of 0.1 km/h it will the front pantograph after 3 seconds with event "PantoUp". The event also starts condition check "PantoDown". When the train is going again and reaches 30 km/h event "PantoDown" is called again. That will lower the front pantograph en starts condition check "PantoUp" again. |
Dit script kan ook gebruikt worden voor AI treinen. Vervang in het script "PlayerEngine" door de ID van de locomotief. |
This script can also be used for AI trains. Replace "PlayerEngine" with the loco's ID in the script. |
Klik hier voor deel 2 van deze tutorial. | Click here for part 2 of this tutorial. |